213 IX: RPi5GPIO: A RPi.GPIO Kludge for Simple RPi 5 Python Code (213.html)
Keywords
ICH180RR RPi5GPIO RPi.GPIO gpiozero "Raspberry Pi 5" "1-character conversion"
"BroadCom GPIO Pin Numbers" GPIO.BCM Python Thonny "Cytron Maker pHAT"
"Maker pHAT" "RPi.GPIO Replacement" Kludge "RPi 5 Python" "RPi 5B" RPi-5B RPi5B
Raspberry "Raspberry Pi 5B" Python3 Test_RPi5GPIO_I_py.txt "Crucial T705" Micron
T705 "T705 Limited Edition"
/KeywordsEnd
(To enlarge .....Click it)
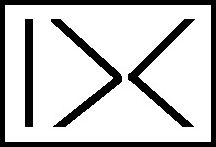
IX or (IX by DC) or "|><"
This article is a part of the IX family of software.
Introduction
******** RPi.GPIO for Raspberry Pi 5B ********
Raspberry Pi 5B computers (unlike previous Raspberry Pi computers) cannot use RPi.GPIO to control simple GPIO pins. Users must import and use gpiozero (or something similar) instead, entailing extensive code conversions. The author of this website has developed an easy method to painlessly convert simple RPi.GPIO code into gpiozero code. This method is useful when converting very simple Python programs that use RPi.GPIO for use on Raspberry Pi 5B computers. Initial testing shows that Python conversion to control GPIO pins on the RPi 5B, requires changing as little as 1 character in the code. ONLY ONE CHARACTER!!
Conversion From RPi.GPIO To gpiozero
The simple Python program shown below used "import RPi.GPIO . ." to control the GPIO pins.
This program was easily converted to use "import RPi5GPIO . ." to control the GPIO pins
on a Raspberry Pi 5B (using gpiozero in RPi5GPIO) as shown below:
Simply replace one "." with a "5"
#Test_RPi5GPIO_D.py
#By D@CC on 2024AJan18
#Purpose: Control GPIO pins using RPi.GPIO code on a RPi 5B
#Source: https://ephotocaption.com/a/213/213.html
import time
PINout=12
#import RPi.GPIO as GPIO #Deprecated # v
import RPi5GPIO as GPIO # <- import RPi5GPIO as GPIO
GPIO.setmode(GPIO.BCM) # ^
GPIO.setup(PINout,GPIO.OUT)
while True:
GPIO.output(PINout,True) #turn LED on
time.sleep(1)
GPIO.output(PINout,False) #turn LED off
time.sleep(1)
#/Test_RPi5GPIO_D.py
BEWARE: RPi5GPIO.py in Source 02 will ONLY handle BroadCom GPIO pin numbers.
A very short (5 sec) video of this blinking blue led can be found in a .MOV in Video Source 01. The RPi 5B is in a 3D case with the Cytron Maker pHAT connected on top along the right side of the video. The green Power-On led of the RPi 5B can be seen on the top edge of the case. The blinking blue led can be seen about 80% down the top of the Cytron Maker pHAT board. To view the .MOV, simply download and play it.
The black and orange case housing the RPi 5B is Printables # 642650 which was recently created for the author by Myrle. (Thank you, Myrle).
RPi5GPIO Issue(s)
ISSUE 01 - as of 2024AJan19 Any statements testing for GPIO.HIGH (or GPIO.LOW) can simply be buffered with the unity() function.
This is really a minor issue because GPIO.input(pin) can be compared to True (not to GPIO.HIGH).
RPi.GPIO vs gpiozero
If a Cytron Maker pHAT (Source 06) is connected to the GPIO pins on a RPi 5B, the LED on GPIO 12 will be controlled by the Test_RPi5GPIO_D.py code in Source 03.
One might wonder which (of RPi.GPIO or gpiozero) is being used when the source code differences are so minimal. One sure way (to distinguish between the two) is to run the above program twice under Thonny on a RPi 4. Run it twice with the "." and then twice again with the "5". When RPi.GPIO is being used, Thonny will produce the warning: "Run Time Warning: This channel is already in use" the second time. When gpiozero is being used (via the "import RPi5GPIO as GPIO"), Thonny will produce no such warning the second time. In all cases, the LED will be blinking, as it should. Of course, when using the "." on the RPi 5, an error will occur because "import RPi.GPIO . . " doesn't work on a Raspberry Pi 5.
On an RPi 5B, the use of RPi.GPIO will produce the error message: "Run Time Error: cannot determine SOC peripheral Base Address". On an RPi 5B, when gpiozero is used (via the "import RPi5GPIO as GPIO" statement) the program will run successfully, the LED on the Cytron Maker pHAT will be blinking. The Raspberry Pi 5B will be blinking the LED even though the Python code "seems" to be using RPi.GPIO statements.
Simply changing one character in the " import RPi.GPIO as GPIO" statement makes the former code (written for RPi.GPIO) work on the Raspberry Pi 5B. It is, of course, the "." in RPi.GPIO that must be changed to a "5".
On the Raspberry Pi 5B, this program can be run in the Terminal using Python with the same results. To function correctly in Terminal mode on the RPi 5B, it is only necessary to "import RPi5GPIO as GPIO" for this program to run. Of course, Source 02 containing RPi5GPIO_py.txt must be downloaded into the folder containing the program that will import it. It will also be necessary to rename RPi5GPIO_py.txt to RPi5GPIO.py . The reason that RPi5GPIO.py is downloaded as RPi5GPIO_py.txt is because any Raspberry computer will immediately run any code using the extension ".py" that is downloaded.
NOTE: As of 2024AJan21, the RPi5GPIO.py code only controls LEDs and push-buttons on the GPIO pins.
Source 01 (vsn I) has been modified to also control a LED using a pushButton. It has been tested on Raspberry Pi computers.
Source 02 (containing the latest RPi5GPIO library) works well. It has been tested on various Raspberry Pi computers.
(To enlarge .....Click it)
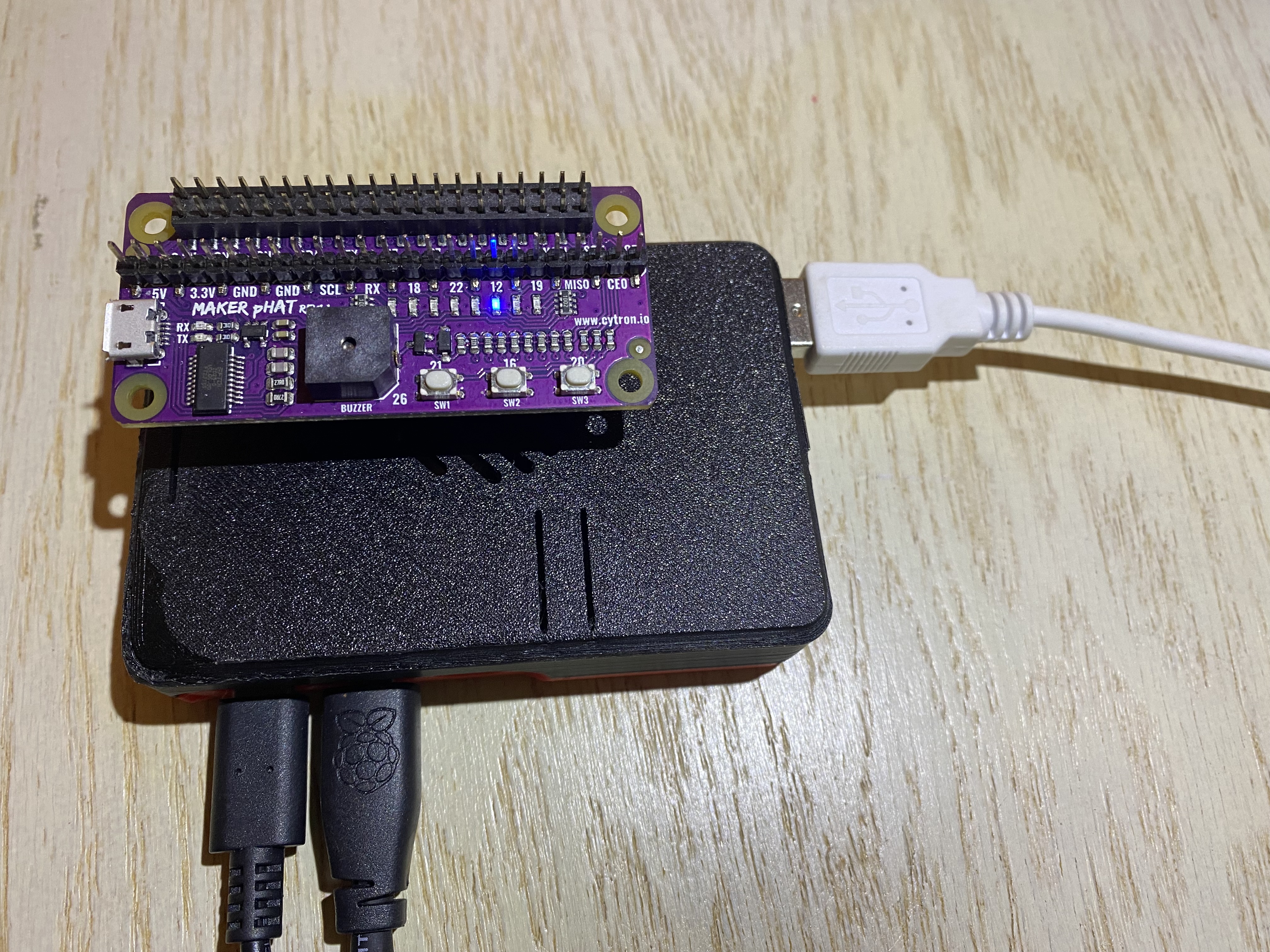
The Cytron Maker pHAT mounted on the RPi 5B in a plastic case
A .MOV of the blinking led can be seen in Video Source 01.
Compute Module 4 GPIO Pin Numbers
The author has recently discovered that the Compute Module 4 only recognizes BroadCom GPIO Pin numbers (not physical GPIO pin numbers). Therefore the author is content that gpiozero does NOT support Physical GPIO Pin numbers, neither will RPi5GPIO. Therefore users must convert such code so that it uses BroadCom GPIO Pin numbers.
Use The Python 3 exec() Function To Manipulate Some gpiozero Statements
Some gpiozero statements are difficult (or perhaps impossible) to create as a string and run without a special function. An example is:
>>> from gpiozero import Button
>>> pinIn = 21
>>> pushButtonA=Button(pinIn,pull_up = True,bounce_time= None)
The 3rd statement above cannot easily be manipulated (as shown below) and then run within the same Python program.
>>> code="pushButtonA=Button(pinIn" + ",pull_up = True,bounce_time= None" + ")"
Fortunately, python3 provides a solution to this problem (see Source 10). It is:
>>> from gpiozero import Button
>>> pinIn = 21
>>> code="pushButtonA=Button(pinIn" + ",pull_up = True,bounce_time= None" + ")"
>>> exec(code)
The 4 lines of python3 code use gpiozero to create the object "pushButtonA" as BroadCom GPIO pin 21 with a pull_up resistor with no handling of bounce_time (no allowance for contact bouncing). This python3 "exec()" function can even handle multiple lines of code. Note that the "import" statement can either precede or be included in the "code" variable. This means that "exec()" handles "code" as a "live" python3 statement, making use of a common pool of variables and functions etc in common with the program that runs the "exec()" function. The variable "pinIn" is defined (and assigned a value) in the main program, but the exec(code) makes use of this exact same variable.
The author's recent attempt to use "exec(code)" in this manner did not succeed, although executing simpler statements were successful.
Overheating the Raspberry Pi 5B
Anyone concerned about overheating the RPi 5B can check its CPU temperature (in Terminal) using:
>$ usr/bin/vcgencmd measure_temp
>$ temp=64.2'C
or
>$ cd Desktop/IX_assets
>:~Desktop/IX_assets $ sh CPU_temp.sh
>$ temp=64.2'C
The Raspberry Pi 5B will automatically throttle the clock speed making an effort to keep the CPU temperature below 85°C. It will also make best use of any available fan or active cooler. To date the author is using a 4GB RPi 5B and an NVMe SSD (on a PiNEBERRY Board) using a feeble power supply (designed for an RPi 4). Minor computing activities, to date, have put no stress on this little power supply, with RPi 5B temperatures remaining below 70°C. Pinouts for the RPi 5B can be found in Source 05. Hopefully they will also publish connector pinouts soon.
Waveshare HAT+ PCIe NVMe SSD board
Source 07 describes a recently announced US$9 HAT+ adapter (SKU: 26583 shown below left) that connects a M.2 NVMe 2230 or 2242 SSD drive to the PCIe connector on the Raspberry Pi 5B. This is the least expensive adapter board of its kind that has been announced to date as of 2024AJan20. This adapter has a big hole directly under the SSD to facilitate RPi 5B cooling by the active cooler that can be positioned between the HAT+ and the RPi 5B (below right). Waveshare describes this adapter as being a PCIe_TO_M.2_HAT+ . It also allows for passage of a camera cable (or 2) down to the RPi 5B. The hold-down bolt (screw) for the SSD is conveniently located on top, facilitating exchange of SSDs.
On 2024AJan20, the author ordered 2 of the HAT+ adapters from WaveShare. On Jan 20, I was notified that they are on backorder. I was informed that the items were shipped today 2024AJan24 and arrived around 2024AJan28. Source 12 provides instructions how to install it.
Four other models of SSD hat adapters for the Raspberry Pi 5 are available from GeekWorm (Source 11). They are slightly more expensive than the WaveShare adapter shown above but can accept longer SSD drives.
Other Thoughts
The Future Crucial T705 SSD: Blistering FAST 14,500 MB/s of Bandwidth by Micron
(To enlarge .....Click it)
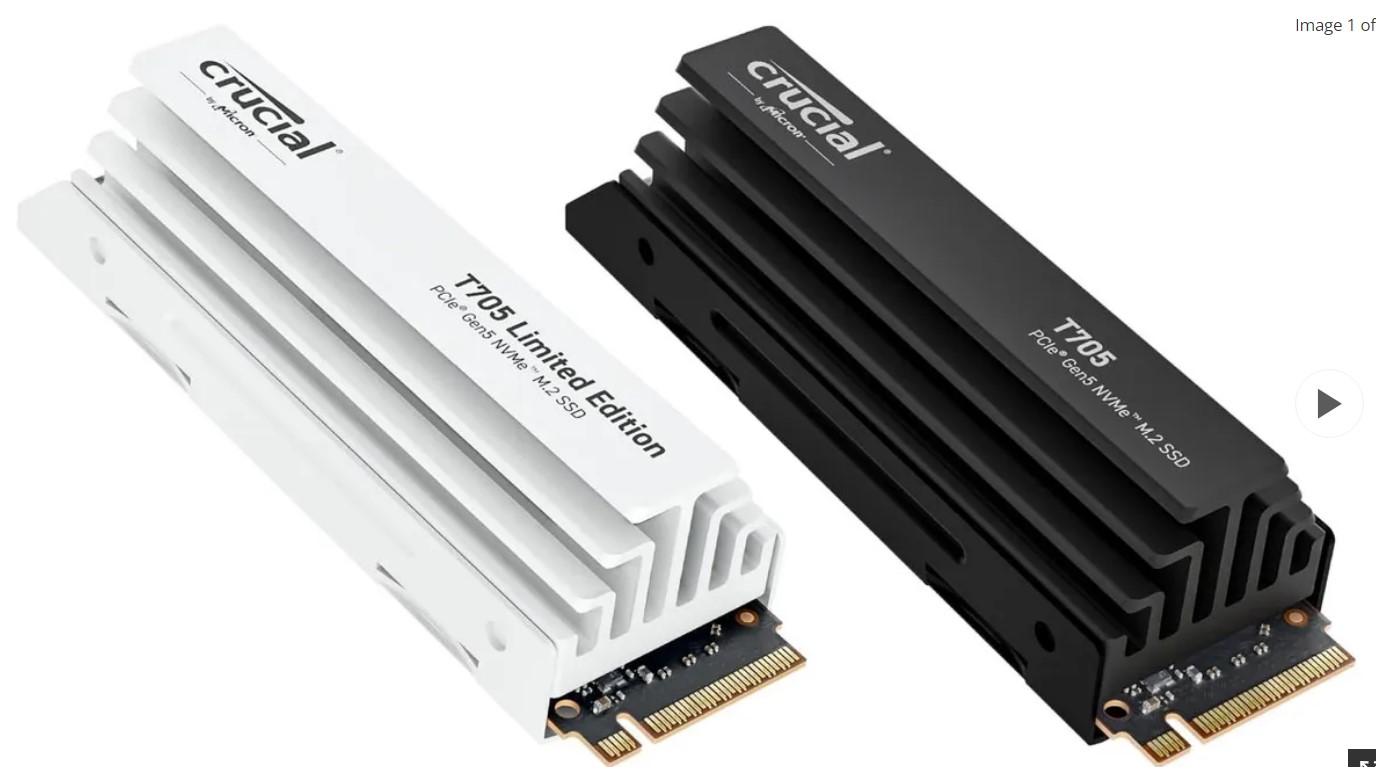
Two devices named Crucial T705
In Source 13, on 2024BFeb06, Tom's Hardware has leaked (from DeepBlue) a very fast PCIe SSD. Not very much is known about the internals of two new T705 CRUCIAL products by Micron. They are both PCIe Gen5 NVMe M.2 SSD named T705. The article in Source 13 provides remarkable speed measurements. Both devices have a PCIe 5.0 x4 interface and capacities of 1,2 4 TB. The T700 read speed is 12,400 MB/s. Tom's Hardware says "The T705 read speed is 14,500 MB/s. Micron / CRUCIAL might well be using the new E26 Max14um controller for the T705." The IOPS numbers are 1,800K and 1,500K. There is also a white T705 Limited Edition of shown in the image above. The T705 Limited Edition has a suffix of SSD5A. Note the massive heat sink on these two model T705 SSDs.
In comparison, the Avg. Sequential Read Speed of a KIOXIA (Toshiba) XG5 NVMe PCIe M.2 256GB SSD is only 1,131 MB/s which is under
10% of the speed of the T705. The T705 PCIe interface is "x4" which is incompatible with the RPi 5B PCIe which is only "x1". The number following the "x" specifies the bit width of the data path of the PCIe.
Ham Radio using the Raspberry Pi
Source 08 will take you to a lengthy article explaining how to use a Raspberry Pi as part of a radio receiver and transmitter. For communicating, a web-browser-compatible SDR receiver (or transceiver such as the "HackRF One") must be attached to the Raspberry Pi. Today's relatively slow computers restrict High Frequency Band usage, so the lower frequency bands are usually favored. As of 2024, Ham Radio Operators still need to pass an exam before going "on-air". The author of this website is a licenced Ham Radio Operator with call sign VE3IAE. Links to distant physical radios via a Web Browser permit communication using the distant antennae!! See Source 09 for my article that describes the use of modern Ham Radio Equipment via the Internet.
In 2024, via the internet, you can "borrow or share" another operator's receiver controlling it yourself. Transmission of the audio for thousands of miles is quite a simple matter for today's world wide web. Even viewing and operating the controls of a distant receiver is easily accomplished. The International Beacon Project (IBP) adds a new dimension to Ham Radio. This project continuously operates beacons (transmitters) in almost every time zone around the world. Anyone can "listen" for these beacons to "see" the communication conditions between various pairs of points all around the world. Such clever beacons were not even thought of when I first became a Ham in 1960. At that time, before the wide-spread use of transistors, in 1960, all of our radios used high-voltage vacuum tubes. Today, most homes have millions of transistors, but no tubes.
Sources
Video Sources
Video Source V213:01: Test_RPi5GPIO_D.py on RPi 5B (0:0:05)
by D@CC on 2024 A Jan 21
Web Sources
Web Source S213:01:www
Test_RPi5GPIO_I.py (as text)
by D@CC on 2024AJan21
Web Source S213:02:www
RPi5GPIO.py (as text)
by D@CC on 2024AJan21
Web Source S213:03:www
Test_RPi5GPIO_D.py (as text)
by D@CC on 2024AJan18
Web Source S213:04:www
gpiozero - migrating from RPI.GPIO
by gpiozero read the docs on 2024AJan19
Web Source S213:05:www
pinout.ai raspberry-pi-5
by FLEETSTACK.IO on 2024AJan19
Web Source S213:06:www
Cytron Maker pHAT
by Cytron as of 2024AJan19
Web Source S213:07:www
Waveshare HAT+ PCIe NVMe SSD
by Brad Linder on 2024AJan19
Web Source S213:08:www
Ham Radio on a Raspberry Pi
by Ham Radio Planet as of 2024AJan19
Web Source S213:09:www
HAM: SDR Amateur Radio (162.html)
by D@CC (VE3IAE) on 2021 E May 17
Web Source S213:10:www
Execute code in a data string
by geeksforgeeks before 2024AJan22
Web Source S213:11:www
GeekWorm X1001 M.2 Key-M NVMe PCIe adapter
by geeksforgeeks before 2024AJan22
Web Source S213:12:www
WaveShare PCIe_TO_M.2_HAT+ adapter
by WaveShare c 2024AJan31
Web Source S213:13:www
Crucial T705: 14,500 MB's
by Mark Tyson of Tom's Hardware on 2024BFeb06
/SourcesEnd
There is a way to "google" any of the part-numbers, words or phrases in all my articles. This "google-like" search limits itself ONLY to my articles. Just go to the top of "Articles by Old King Cole" and look for the "search" input box named "freefind".
Date Created:2024 A Jan 17
Last Updated:2024 B Feb 06
All rights reserved 2024 by © ICH180RR
saved in E:\E\2022\DevE\MyPagesE\Globat\ePhotoCaption.com\a\213\213.html
backed up to ePhotoCaption.com\a\213\213_2024AJan19.html
Font: Courier New 10 (monospaced)
/213.html